Flutter Development
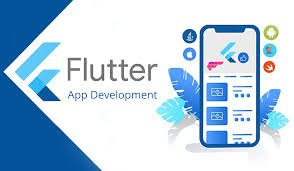
Flutter is a popular open-source UI framework developed by Google, primarily used for building natively compiled applications for mobile, web, and desktop from a single codebase. It uses the Dart programming language, which is also developed by Google. Flutter is known for its performance, expressive UI, and ease of use.
Here are the key features of Flutter development:
1. Single Codebase for Multiple Platforms
With Flutter, you can write a single codebase that can run on iOS, Android, web, and even desktop platforms (macOS, Linux, and Windows). This is a major advantage as it reduces development time and costs.
2. Hot Reload
Flutter comes with a “hot reload” feature that allows developers to instantly see the changes made in the code without losing the current state of the application. This makes it very useful for testing and iterating quickly.
3. Customizable Widgets
Flutter provides a wide variety of widgets, which are the building blocks of any Flutter application. These widgets are highly customizable, and you can build complex UIs with ease.
4. Performance
Flutter apps are compiled to native machine code, which gives them excellent performance compared to other cross-platform frameworks like React Native, which rely on JavaScript bridges. Flutter’s performance is on par with native applications.
5. Rich Ecosystem
Flutter has a large and active community, and the ecosystem is rapidly growing. It offers a wide range of plugins and packages that can be easily integrated into your app.
6. Material Design & Cupertino Widgets
Flutter supports Google’s Material Design for Android apps and Apple’s Cupertino widgets for iOS apps, which means you can create apps that feel native to both platforms.
Basic Steps to Get Started:
- Install Flutter
You can install Flutter on Windows, macOS, or Linux. You’ll also need to set up an editor, like Visual Studio Code or Android Studio, and install the Dart plugin.- Download Flutter: Flutter Install Guide
- Install dependencies: Xcode (macOS) for iOS, Android Studio for Android.
- Create a New Flutter Project
Open the terminal and run the following command to create a new project:bashflutter create my_flutter_app
- Run Your Flutter App
After creating a new Flutter project, you can navigate to the project directory and run the app:bashcd my_flutter_app
flutter run
- Edit Your App
In thelib/main.dart
file, you can start modifying your app. This file is the entry point for your app. - Use Widgets
Flutter’s UI is built using widgets. Every element on the screen is a widget, including text, buttons, and images.
Example of a Simple Flutter App:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘Flutter App’)),
body: Center(child: Text(‘Hello, Flutter!’)),
),
);
}
}
Additional Resources:
- Flutter Documentation: Flutter Docs
- Dart Programming Language: Dart Docs
- Flutter Packages: Flutter Packages